Dropdown
Workflow
This element works similarly to the native dropdown in terms of events, but has many additional functions as well as an easy o use preset system. In order to change the dropdown item preset, you can simply select the ‘Item Preset’ prefab and make changes.
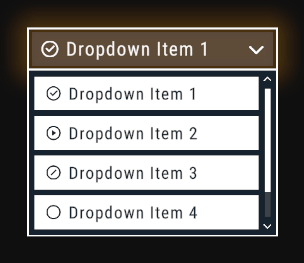
Localization
In order to use the localization system with dropdowns, you can select the object and assign a valid localization key for each dropdown item. Everything will be handled automatically as long as the localization system is active.
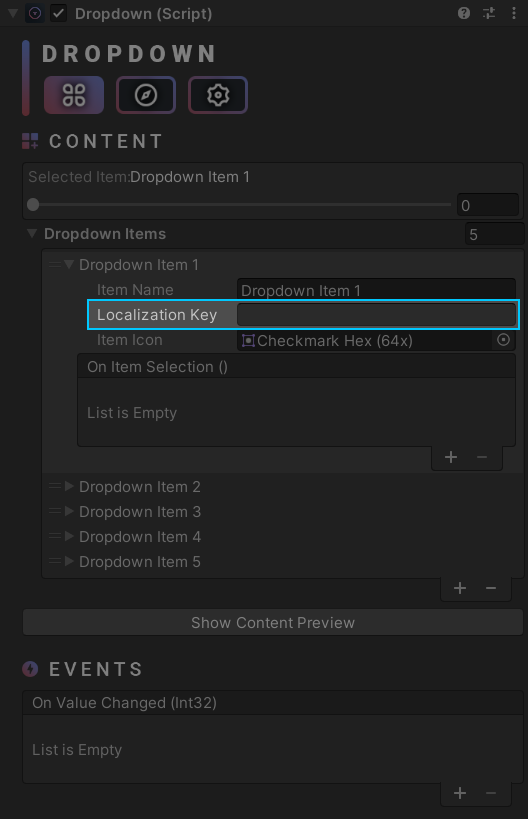
Scripting
using UnityEngine;
using Michsky.UI.Heat; // Heat UI namespace
public class SampleClass : MonoBehaviour
{
// You may have to specify Michsky.UI.Reach in some cases
[SerializeField] private Michsky.UI.Reach.Dropdown dropdown;
void Start()
{
// Sets the dropdown index and updates the layout
dropdown.SetDropdownIndex(0);
// Process the event based on the given item index
dropdown.onValueChanged.Invoke(0);
// Register a new dynamic event
dropdown.onValueChanged.AddListener(TestFunction);
// Set the interaction state
dropdown.Interactable(true);
// Create a new item
dropdown.CreateNewItem("Item Name");
// Create a new item but do not initialize the component
// Use this with (e.g. for, foreach)
dropdown.CreateNewItem("Item Name", false);
// Remove the item
dropdown.RemoveItem("Item Name");
// Remove the item but do not initialize the component
// Use this with loops (e.g. for, foreach)
dropdown.RemoveItem("Item Name", false);
// Initialize the component
// Use this after creating or removing items within a loop
dropdown.Initialize();
}
void TestFunction(int value)
{
Debug.Log("Dropdown item selected: " + value);
}
}
How can we help?
A premium WordPress theme with an integrated Knowledge Base,
providing 24/7 community-based support.