Messaging
Workflow
Functional messaging app which supports getting and sending messages at runtime.
Messaging app depends on its manager and scriptable objects. In order to add a new chat, you have to create a new chat asset from Assets > Create > DreamOS > New Messaging Chat. You can now select Canvas > Managers > Messaging and add hit add a new chat, and then assign the asset you’ve created. Each item has its own unique title and person data. All of the messages in the chat asset will be generated at runtime.
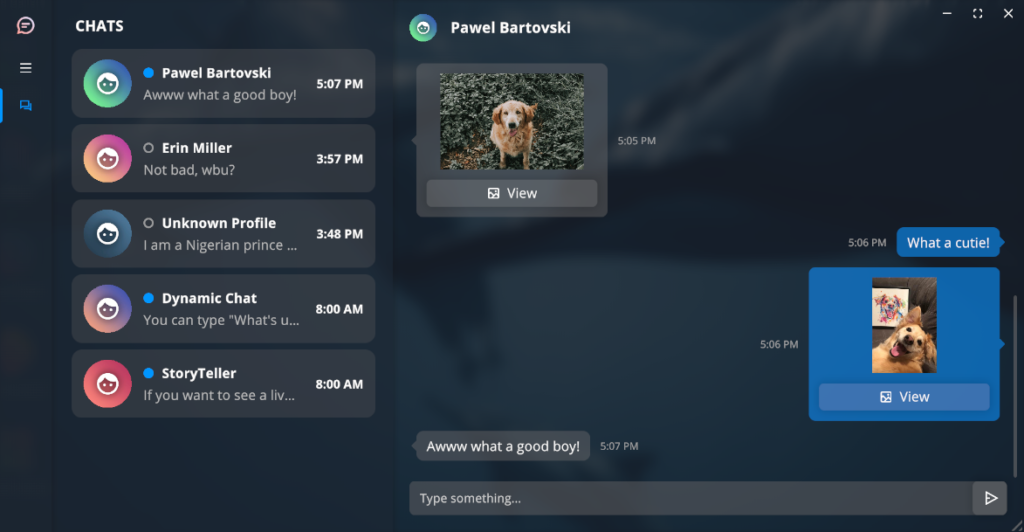
Save Message History
It is possible to save sent messages at runtime. You can select the ‘Messaging Manager’ component, open the settings tab, and enable the ‘Save Message History’ option. Afterwards, make sure to add and assign the ‘Message Storing’ component.
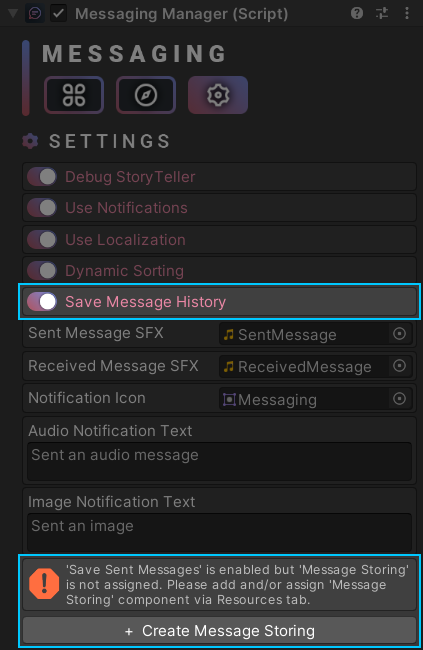
Scripting
using UnityEngine;
using Michsky.DreamOS; // DreamOS namespace
public class SampleClass : MonoBehaviour
{
[SerializeField] private MessagingManager messagingApp;
void YourFunction()
{
// Force chat data to be updated
messagingApp.Initialize();
// Get layoutIndex from chat title
int layoutIndex = messagingApp.GetChatLayoutIndexFromTitle("Chat Title");
// Instantiate standard messages - you can leave parent as null and set a layoutIndex instead
messagingApp.CreateMessage(layoutIndex, "Message content");
messagingApp.CreateIndividualMessage(layoutIndex, "Message content");
// Instantiate date indicator - you can leave parent as null and set a layoutIndex instead
messagingApp.CreateDate(layoutIndex, "21 Jul, 2020");
// Instantiate image message - you can leave parent as null and set a layoutIndex instead
messagingApp.CreateImageMessage(layoutIndex, Sprite sprite, "Title", "Description");
messagingApp.CreateIndividualImageMessage(layoutIndex, Sprite sprite, "Title", "Description");
// Instantiate audio message - you can leave parent as null and set a layoutIndex instead
messagingApp.CreateAudioMessage(layoutIndex, AudioClip audio);
messagingApp.CreateIndividualAudioMessage(layoutIndex, AudioClip audio);
// Create storyteller
messagingApp.CreateStoryTeller("Message/Chat ID", "StoryTeller Item ID");
// Enable or disable specific dynamic message reply
messagingApp.EnableDynamicMessageReply("Message ID");
messagingApp.DisableDynamicMessageReply("Message ID");
// Allow or disallow input submit
messagingApp.AllowInputSubmit(true);
// Change persona status
messagingApp.ChangeStatus(MessagingManager.Status.Offline, "Chat Title");
}
}
How can we help?
A premium WordPress theme with an integrated Knowledge Base,
providing 24/7 community-based support.